The Qt framework is a powerful tool for C++ application development, offering a wide range of features for creating graphical user interfaces (GUIs), file manipulation, networking, and more. In this post, we’ll cover the basic concepts of Qt, from installing and configuring the development environment to creating a simple “Hello World” application using C++ and Qt.
1. What is Qt?
Qt is a cross-platform framework that enables the development of high-quality graphical user interface applications. With Qt, you can develop applications that run on Windows, macOS, Linux, Android, iOS, and other platforms from a single codebase.
2. Installing and Configuring the Development Environment
To start developing with Qt, we need to install Qt Creator, an integrated development environment (IDE) specifically designed to work with Qt. Here are the installation steps:
Step 1: Downloading Qt Creator
- Go to the official Qt website: https://www.qt.io/download.
- Download the Qt installer for your operating system.
Step 2: Installing Qt Creator
- Run the downloaded installer.
- Follow the installation wizard steps.
- During installation, select the necessary components, such as Qt 5.15.x and Qt Creator.
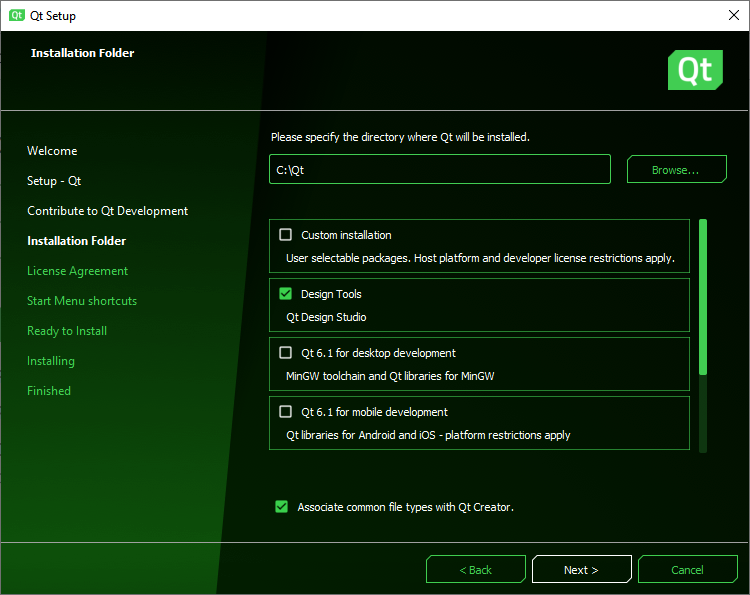
Step 3: Configuring Qt Creator
- After installation, open Qt Creator.
- Go to “Tools” > “Options”.
- In “Kits”, ensure that the Qt version you installed is configured correctly.
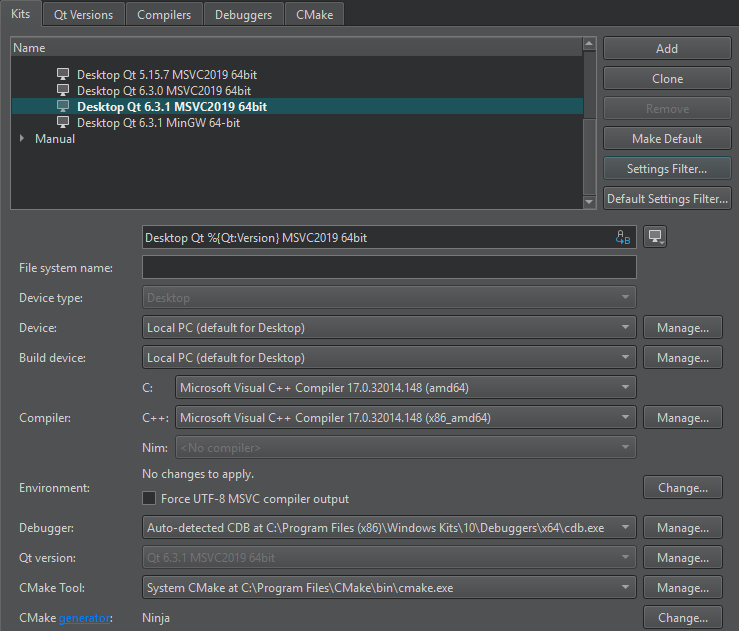
3. Creating a “Hello World” Project in C++ with Qt
Now let’s create a simple “Hello World” program using Qt.
Step 1: Creating a New Project
- Open Qt Creator.
- Select “File” > “New File or Project”.
- Choose “Application” > “Qt Widgets Application” and click “Choose…”.
- Name your project and choose the directory where it will be saved.
- Click “Next” and select the Qt version configured earlier.
- Click “Finish”.
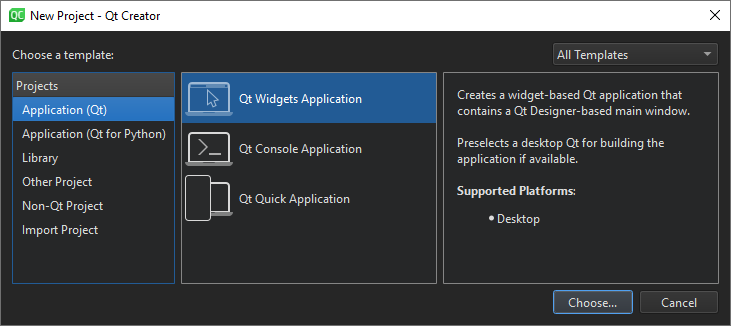
Step 2: Editing the Source Code
- In Qt Creator, navigate to the
main.cpp
file. - Replace the existing code with the following:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button(“Hello World”);
button.show();
return app.exec();
}
Step 3: Building and Running the Project
- Click the play icon (Run) in the toolbar.
- Qt Creator will compile and run your project, displaying a window with a “Hello World” button.
Conclusion
Qt is an extremely powerful and versatile tool for C++ application development. With this introduction, you have learned how to install Qt, configure the development environment, and create your first “Hello World” program. In future posts, we will explore more features of Qt and how to use them to develop more complex applications.
I hope this introduction to Qt has been helpful.
With this post, you’ll be ready to start your journey with Qt and explore all its possibilities in application development.
Happy coding!
If you have any questions or suggestions, feel free to leave a comment below.
Until next time!
Stay Connected!
Follow me on LinkedIn for more content on software development and emerging technologies: LinkedIn.
C++ #Qt #SoftwareDevelopment #Programming #HelloWorld